Input/output options
Using opt fields to customize API responses
Quick start
For more examples of how to build API requests with input/output options, visit the quick start guide.
Options
Some requests return "compact" representations of objects in order to conserve resources and complete the request more efficiently. Other times, requests return more information than you may need. Using input/output options allows you to list the exact set of fields that the API should return for the objects.
Usage
In addition to providing fields and their values in a request, you may also specify options
to control how your request is interpreted and how the response is generated.
For GET
requests, options are specified as URL parameters prefixed with opt_
:
?opt_pretty=true
?opt_fields=followers,assignee
For POST
or PUT
requests, options are specified in the request body:
options: {
pretty: true,
fields: ["followers", "assignee"]
}
- If the body uses the
application/x-www-form-urlencoded
content type, then options are prefixed withopt_
, just like forGET
requests. - If the body uses the
application/json
content type, then options are specified inside the top-leveloptions
object (i.e., a sibling of thedata
object).
These options can be used in combination in a single request, though some of them may conflict in their impact on the response.
Option | Description |
---|---|
pretty | Provides the response in "pretty" output. In the case of JSON this means doing proper line breaking and indentation to make it readable. This will take extra time and increase the response size so it is advisable only to use this during debugging. |
fields | Some requests return compact representations of objects, to conserve resources and complete the request more efficiently. Other times requests return more information than you may need. This option allows you to list the exact set of fields that the API should be sure to return for the objects. The field names should be provided as paths, described below. The gid of included objects will always be returned, regardless of the field options. |
Example
Here is an example request to get a user:
curl --request GET \
--url 'https://app.asana.com/api/1.0/users/me?opt_fields=name,email&opt_pretty=true' \
--header 'accept: application/json' \
--header 'authorization: Bearer YOUR_TOKEN_HERE'
Note that in the URL, we appended opt_fields=name,email&opt_pretty=true
. This should yield both the name
and email
of the user in the response. This response will also be "pretty" (i.e., formatted JSON), as a result of the true
value for opt_pretty
.
Here is the response:
{
"data": {
"gid": "12345",
"email": "[email protected]",
"name": "Example User"
}
}
Which opt_fields
are available?
opt_fields
are available?If opt_fields
are available for an API request, you can confirm which valid fields can be included in that request by visiting the response object's schema page in the API reference (e.g., the full schema for tasks).
Alternatively, while viewing an endpoint (e.g., GET /goals) in the API reference, you may also review the enumerated values listed in the opt_fields
section. Consider the following example of including opt_fields
to get more data:
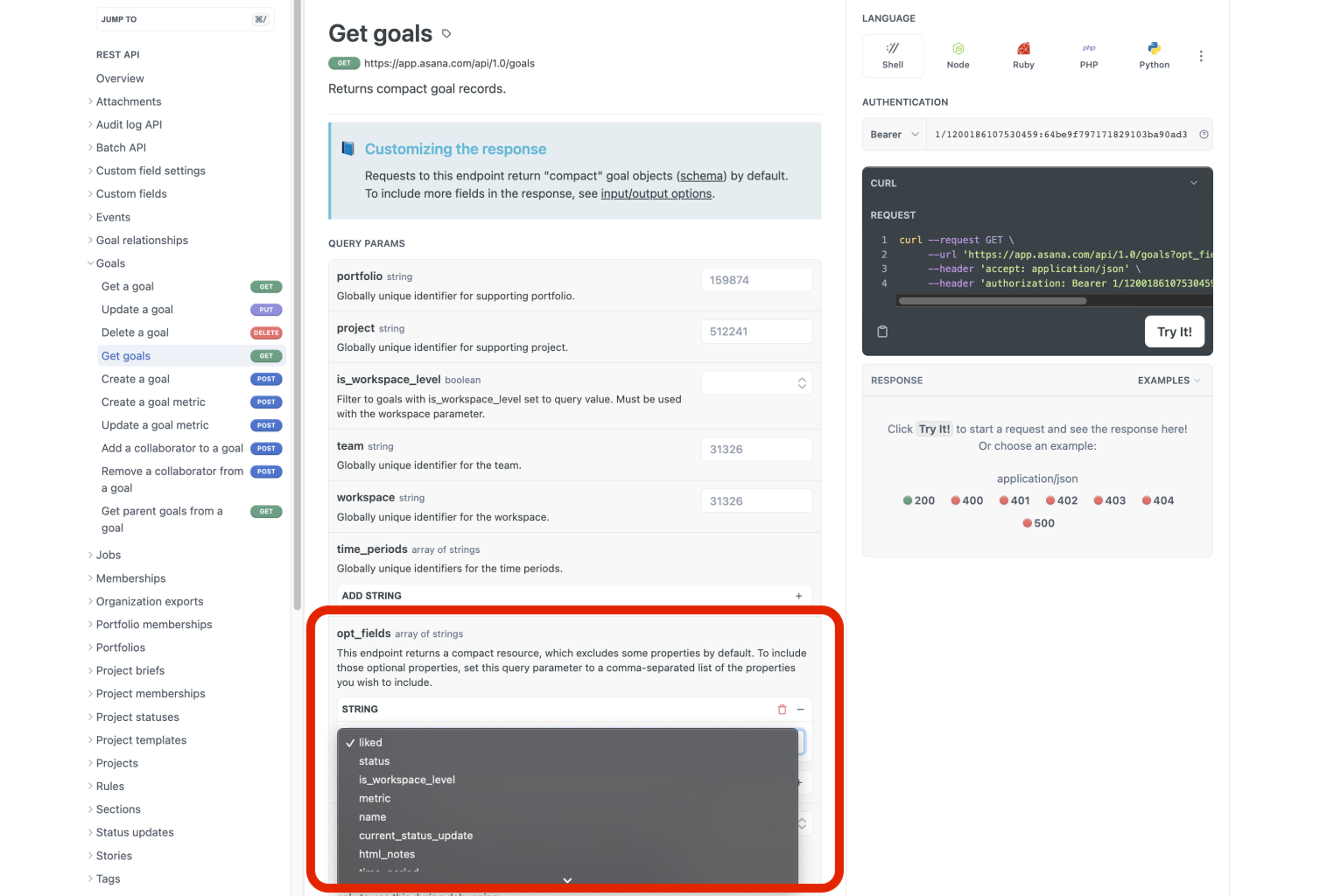
In the above example, enumerated values such as liked
, status
, is_workspace_level
, etc. can be included as opt_fields
values in the request. This allows you to customize the response you receive from the API.
Selecting nested fields
Some output options allow you to reference fields of objects to include in the response. The way to specify a field is by path. A path is made up of a sequence of terms separated by the dot (.
) operator. It takes the form this.a.b
… where this refers to an object returned at the top level of the response, a the name of a field on a root object, b a field on a child object referred to by a, and so on.
For example, when retrieving a task or tasks, the path this.followers.email
refers to the email
field of all users
mentioned in the followers
field of the task or tasks returned. Here's the annotated output:
"data": { < this
"gid": "1001",
"name": "Feed the cat", < this.name
"workspace": { < this.workspace
"gid": "14916",
"name": "Shared Projects", < this.workspace.name
},
"followers": [{ < this.followers
"gid": "1234",
"email": "tbizarro@…", < this.followers.email
}, {
"gid": "5678",
"email": "gsanchez@…", < this.followers.email
}]
}
There are also some advanced operators you can use for shorter syntax in selecting fields:
(
.. |
.. )
The group operator can be used in place of any whole term in a path, and will match any of a group of terms. For example:
this.(followers|assignee).email
will match the email
field of the assignee
object or any of the followers
.
Updated about 2 months ago