Migration guide: v5
Migrating from v3 to v5 of the Python library
Introduction
As the Asana API continues to evolve, the Python library must also adapt to ensure users have the best tools for integrating with Asana. This migration guide outlines the key advantages of the newest version of the library (v5.x.x as of this writing), highlights differences between the latest and previous major versions, and offers insights into when and why you may want to consider upgrading.
With the v5 library, users can enjoy several enhancements. For instance, it benefits from automation that enables more frequent updates, allowing access to the latest features and endpoints shortly after their release. Additionally, v5 leverages native implementations for improved compatibility with modern Python environments, eliminating the need for under-the-hood workarounds seen in earlier versions. This makes it more user-friendly, especially for developers familiar with other API libraries, as it is built using industry-standard tools.
Differences between v3 and v5
To facilitate your migration process, our API reference that highlights the differences in syntax between v3 and v5 for each endpoint. Upon selecting Python, the API reference features two tabs for easy comparison: one for v3 syntax and another for v5 syntax.
Here is an example for the POST /tasks endpoint:
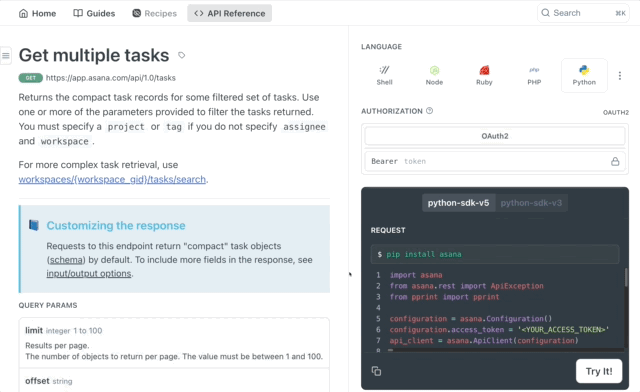
These comparisons are designed to streamline your migration by providing clear examples and helping you quickly adapt your code.
As you transition from v3 to v5 of the Python Asana library, it’s important to understand the fundamental changes that enhance usability and performance. The following are key takeaways that highlight the differences between the two versions:
Client initialization
Client initialization in v5 of the library improves modularity and readability by separating configuration details from API client instantiation, making the code cleaner and easier to manage. This structured approach enhances flexibility, allowing for easier adjustments to settings and ensuring consistency across endpoint usage.
v3 uses asana.Client.access_token()
. Note that the token is directly passed in the client initialization.
asana.Client.access_token()
. Note that the token is directly passed in the client initialization.client = asana.Client.access_token('PERSONAL_ACCESS_TOKEN')
v5 creates a Configuration
object and an ApiClient
, in which the access token is set in a Configuration
object.
Configuration
object and an ApiClient
, in which the access token is set in a Configuration
object.configuration = asana.Configuration()
configuration.access_token = '<PERSONAL_ACCESS_TOKEN>'
api_client = asana.ApiClient(configuration)
Class instantiation
Class instantiation in v5 enhances clarity and organization by creating specific object class instances (e.g., UsersApi
), which encapsulate related methods and functionality. This approach promotes better code structure, making it easier to navigate and understand the library's capabilities. Additionally, it facilitates a more intuitive experience, as each API class can be tailored with its own settings and methods, improving maintainability and usability.
v3 directly calls methods on the client
object:
client
object:result = client.users.get_user(user_gid)
v5 creates an instance of the specific object class (UsersApi
) to access endpoints:
UsersApi
) to access endpoints:users_api_instance = asana.UsersApi(api_client)
api_response = users_api_instance.get_user(user_gid, opts)
Query params
In v3, the fields
parameter is used to specify which opt fields to include in the API response directly within the method call. In v5, this functionality is achieved using the opt_fields
parameter within an options dictionary, allowing for a clearer distinction between different types of parameters and improving code organization. This change enhances readability and makes it easier to manage and modify the request options.
v3 passes query params into the fields
parameter:
fields
parameter:import asana
from pprint import pprint
client = asana.Client.access_token('<PERSONAL_ACCESS_TOKEN')
result = client.tasks.get_tasks({'assignee': 'me', 'workspace': '<WORKSPACE_GID>'}, fields='assignee,workspace,completed,completed_by', opt_pretty=True)
for task in result:
pprint(task)
v5 passes query params directly through the opts
dictionary:
opts
dictionary:import asana
from asana.rest import ApiException
from pprint import pprint
configuration = asana.Configuration()
configuration.access_token = '<PERSONAL_ACCESS_TOKEN>'
api_client = asana.ApiClient(configuration)
tasks_api_instance = asana.TasksApi(api_client)
opts = {
'assignee': "me",
'workspace': "<WORKSPACE_GID>",
'opt_fields': 'assignee,workspace,completed,completed_by'
}
try:
tasks = tasks_api_instance.get_tasks(opts)
for task in tasks:
pprint(task)
except ApiException as e:
print("Exception when calling TasksApi->get_tasks: %s\n" % e)
Pagination
Both v3 and v5 of the library support pagination by default, but there is a key difference in their configurations: v5 has a default limit of 100
items per page, compared to 50
in v3 (see the updated configuration here).
Rate limits
This shift from 50 to 100 items per page significantly impacts API usage and efficiency. In earlier versions, a default page size of 50 meant that fetching a large dataset—such as 1,000 tasks—required 20 API requests. This higher number of requests could lead to quicker hits against API rate limits, as multiple calls are made in succession, potentially resulting in throttling.
With the updated v5 library, however, the same request for 1,000 tasks can be fulfilled in just 10 API calls. This reduction in the number of requests not only decreases the likelihood of exceeding rate limits, but also minimizes the overhead associated with multiple calls. Overall, this optimization streamlines data retrieval and enhances the API interaction experience, making it smoother for users working with larger datasets.
Ending pagination early
Similar to previous version of the library, in scenarios where you want to end pagination early, you can specify an item_limit
in the method call. This will stop the iterator from going past that limit. This item_limit
is largely passed in the same way as a parameter in both v3 and v5 of the library.
Here are examples for the same GET /projects/{project_gid}/tasks request with an item_limit
of 3
:
import asana
client = asana.Client.access_token('PERSONAL_ACCESS_TOKEN')
result = client.tasks.get_tasks_for_project(<PROJECT_ID>, {}, item_limit=3)
import asana
from asana.rest import ApiException
from pprint import pprint
configuration = asana.Configuration()
configuration.access_token = '<PERSONAL_ACCESS_TOKEN>'
api_client = asana.ApiClient(configuration)
tasks_api_instance = asana.TasksApi(api_client)
project_gid = "<PROJECT_ID>"
opts = {}
try:
api_response = tasks_api_instance.get_tasks_for_project(project_gid, opts, item_limit=3)
for data in api_response:
pprint(data)
except ApiException as e:
print("Exception when calling TasksApi->get_tasks_for_project: %s\n" % e)
Disabling the default pagination behavior
For details on disabling the default pagination behaviors of the library, see this section of the README in python-asana on GitHub.
Upgrading
Note that upgrading is not mandatory. You can continue using the previous version of the Python client library. However, be aware that v3 will not receive new features as the Asana API evolves, which may limit your access to future improvements.
When to upgrade
Deciding to upgrade your library version is crucial and should align with your application's needs. Here are some scenarios to consider:
- Regularly updated applications: If your application frequently adds new features, upgrading to v5 can streamline future updates and ensure access to the latest Asana functionalities
- New development projects: For new projects, it’s advisable to use the latest library version from the start. This helps you leverage recent features and bug fixes, enhancing the development process
By assessing these factors, you can make an informed decision about whether upgrading the library is right for your application.
How to upgrade
Upgrading to the latest version of the Python Asana library is simple. You can easily install the latest version using pip
. If you already have the library installed, just run the following command in your terminal to facilitate the upgrade:
pip install --upgrade asana
This command will ensure that you have the most recent features and improvements available in your project.
Additional resources
Updated 9 months ago